Our provided schematic shows the wiring arrangement for interfacing the microcontroller and motor driver to control the motors. The user-friendly printed circuit board (PCB) design streamlines the setup process, requiring only the connection of power and motor wires for seamless integration. This thoughtful approach prioritizes user convenience while ensuring a smooth and professional experience in deploying our technology across diverse applications.
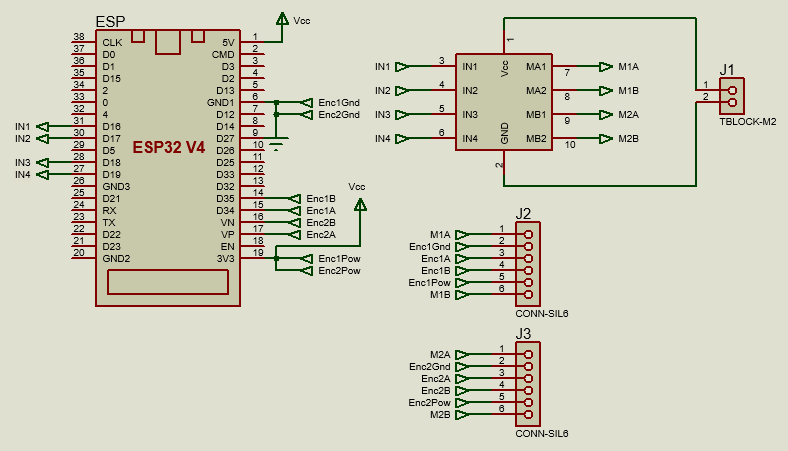
This code serves as the essential programming instructions to enable precise control of the motors through the microcontroller and motor driver. It’s user-friendly, allowing you to customize the motor operations to suit your specific requirements. Feel free to download the complete code or simply copy the snippet for a quick start.
#include "BluetoothSerial.h"
BluetoothSerial SerialBT;
const int Motor1_InA = 19;
const int Motor1_InB = 18;
const int Motor1_EncA = 36; // yellow C1
const int Motor1_EncB = 39; // green C2
const int Motor2_InA = 16;
const int Motor2_InB = 17;
const int Motor2_EncA = 34; // yellow C1
const int Motor2_EncB = 35; // green C2
volatile long motor1EncVal = 0;
volatile long motor2EncVal = 0;
volatile int motorAEncPinALastState = LOW;
volatile int motorAEncPinAState = LOW;
volatile int motorBEncPinALastState = LOW;
volatile int motorBEncPinAState = LOW;
int CurrentMode = 0;
void motor1EncoderISR() {
int b = digitalRead(Motor1_EncB);
if(b > 0){
motor1EncVal++;
}
else{
motor1EncVal--;
}
}
void motor2EncoderISR() {
int b = digitalRead(Motor1_EncB);
if(b > 0){
motor2EncVal++;
}
else{
motor2EncVal--;
}
}
int ControlState = 0;
void setup() {
SerialBT.begin("PixRover1"); // give Bluetooth name
Serial.begin(115200);
pinMode(Motor1_EncA, INPUT);
pinMode(Motor1_EncB, INPUT);
pinMode(Motor2_EncA, INPUT);
pinMode(Motor2_EncB, INPUT);
pinMode(Motor1_InA, OUTPUT);
pinMode(Motor1_InB, OUTPUT);
pinMode(Motor2_InA, OUTPUT);
pinMode(Motor2_InB, OUTPUT);
attachInterrupt(digitalPinToInterrupt(Motor1_EncA), motor1EncoderISR, RISING);
attachInterrupt(digitalPinToInterrupt(Motor2_EncA), motor2EncoderISR, RISING);
}
void loop() {
CheckSerial();
CheckSerialBT();
}
void MoveStop(){
analogWrite(Motor1_InA, 0);
analogWrite(Motor2_InA, 0);
analogWrite(Motor1_InB, 0);
analogWrite(Motor2_InB, 0);
delay(200);
CurrentMode = 0;
}
void MoveForward(uint8_t SpeedA, uint8_t SpeedB){
if (CurrentMode == 0){
analogWrite(Motor1_InA, SpeedA);
analogWrite(Motor2_InA, SpeedB);
}
else{
analogWrite(Motor1_InB, 0);
analogWrite(Motor2_InB, 0);
delay(200);
analogWrite(Motor1_InA, SpeedA);
analogWrite(Motor2_InA, SpeedB);
CurrentMode = 1;
}
}
void MoveBackward(uint8_t SpeedA, uint8_t SpeedB){
if (CurrentMode == 0){
analogWrite(Motor1_InB, SpeedA);
analogWrite(Motor2_InB, SpeedB);
}
else{
analogWrite(Motor1_InA, 0);
analogWrite(Motor2_InA, 0);
delay(200);
analogWrite(Motor1_InB, SpeedA);
analogWrite(Motor2_InB, SpeedB);
CurrentMode = 2;
}
}
void MoveRight(uint8_t SpeedA, uint8_t SpeedB){
if (CurrentMode == 0){
analogWrite(Motor1_InB, SpeedA);
analogWrite(Motor2_InA, SpeedB);
}
else{
analogWrite(Motor1_InA, 0);
analogWrite(Motor2_InB, 0);
delay(200);
analogWrite(Motor1_InB, SpeedA);
analogWrite(Motor2_InA, SpeedB);
CurrentMode = 3;
}
}
void MoveLeft(uint8_t SpeedA, uint8_t SpeedB){
if (CurrentMode == 0){
analogWrite(Motor1_InA, SpeedA);
analogWrite(Motor2_InB, SpeedB);
}
else{
analogWrite(Motor1_InB, 0);
analogWrite(Motor2_InA, 0);
delay(200);
analogWrite(Motor1_InA, SpeedA);
analogWrite(Motor2_InB, SpeedB);
CurrentMode = 4;
}
}
void CheckSerial(){
if (Serial.available()){
int inByte = Serial.read();
if (inByte == 97){ // 97 = a
ControlState = 1;
}
}
}
void CheckSerialBT(){
if (SerialBT.available() >= 1) { // Ensure at least 1 byte is available
char ReceivedChar = SerialBT.read();
if (ReceivedChar == 'S') {
MoveStop();
}
else{
if (SerialBT.available() >= 2) { // Ensure at least 2 more bytes are available
uint8_t ReceivedSpdA = SerialBT.read();
uint8_t ReceivedSpdB = SerialBT.read();
if (ReceivedSpdA != 0 && ReceivedSpdB != 0){
if(ReceivedChar == 'F'){
MoveForward(ReceivedSpdA, ReceivedSpdB);
}
else if(ReceivedChar == 'B'){
MoveBackward(ReceivedSpdA, ReceivedSpdB);
}
else if(ReceivedChar == 'L'){
MoveLeft(ReceivedSpdA, ReceivedSpdB);
}
else if(ReceivedChar == 'R'){
MoveRight(ReceivedSpdA, ReceivedSpdB);
}
else{ SerialBT.println("Error: Unknown Command."); }
}
else{ SerialBT.println("Error: Invalid Value."); }
}
else { SerialBT.println("Error: Insufficient data received."); }
}
}
}
The dart 3D files could be found here